Version Control Best Practices for Custom Software Development
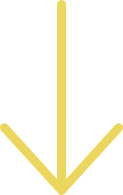
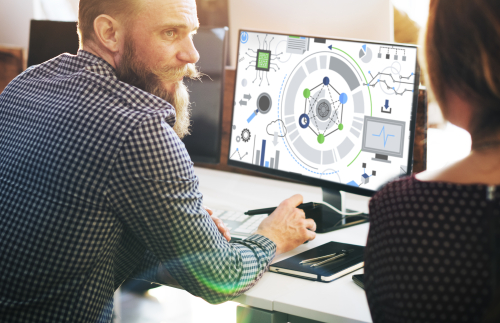
In custom software development, managing code changes efficiently is crucial for maintaining quality and ensuring smooth collaboration among team members. Version control systems (VCS) like Git have become an essential part of the software development process, enabling developers to track, manage, and collaborate on code changes with ease. However, using version control effectively requires more than just committing code—it involves adhering to best practices that streamline workflows, reduce errors, and maintain the integrity of the codebase. This article explores key strategies and best practices for managing version control in a collaborative software development environment.
1. Using Git for Code Tracking and Management
Git is the most widely used version control system for custom software development due to its speed, flexibility, and robust features. Git allows developers to track code changes, collaborate with team members, and manage versions without conflicts. A strong foundation in Git commands and workflows is essential for any development team.
Key Git Concepts:
- Repository (Repo): A central location where code is stored, usually in the cloud (e.g., GitHub, GitLab, Bitbucket).
- Commit: A snapshot of the code at a specific point in time.
- Branch: A parallel version of the code that allows developers to work on features or fixes without affecting the main codebase.
- Merge: The process of integrating changes from one branch into another.
- Rebase: A method of reapplying commits on top of another base tip, ensuring a cleaner commit history.
By using Git to track code changes, teams can easily revert to earlier versions, manage conflicts, and maintain an organized history of code updates. It also provides a way for teams to collaborate on large-scale projects without stepping on each other’s toes.
2. Crafting Clear Commit Messages
One of the simplest but most effective practices for maintaining a clean and understandable codebase is crafting clear and descriptive commit messages. A well-written commit message provides context for the changes made, making it easier for team members to understand the purpose of the commit and track the progress of the project.
Best Practices for Commit Messages:
- Be Descriptive: Avoid generic messages like “fixed bug” or “updated code.” Instead, explain what was done and why. For example, “Fixed issue with user authentication when resetting password.”
- Use the Imperative Mood: Git commit messages are traditionally written in the imperative mood (e.g., “Fix bug” instead of “Fixed bug”). This creates a consistent style and aligns with Git’s internal conventions.
- Limit Line Length: Keep lines of the commit message under 72 characters to ensure they’re readable in Git logs.
- Include References to Issue Numbers: If the commit addresses a specific bug or feature request, include the issue number in the message (e.g., “Fixes #123: Improve search functionality”).
By adhering to these practices, the commit history becomes a valuable tool for tracking changes and understanding the rationale behind each update.
3. Maintaining a Clean Commit History
A clean commit history is vital for understanding the evolution of a project. It allows developers to track the development process, identify when bugs were introduced, and quickly locate relevant changes. To maintain a clean commit history, consider these best practices:
Strategies for Clean Commit History:
- Squash Commits: Instead of making multiple small commits for related changes, squash them into a single, logical commit. This reduces clutter and improves the clarity of the commit history.
- Rebase Before Merging: Rebasing before merging ensures that the commit history remains linear, reducing the risk of merge conflicts and simplifying the review process.
- Avoid “WIP” (Work in Progress) Commits: While it might be tempting to commit frequently with vague messages like “WIP,” this creates unnecessary noise in the history. Use feature branches for ongoing work and commit only meaningful updates.
A clean commit history not only enhances team collaboration but also makes future debugging and feature development easier.
4. Branching Strategies: GitFlow and Feature Branching
Branching is one of Git’s most powerful features, allowing developers to isolate new features, bug fixes, and experiments from the main codebase. This enables teams to work concurrently on different tasks without interfering with one another’s work. A well-defined branching strategy is crucial for managing complex projects with multiple developers.
Popular Branching Strategies:
- GitFlow: GitFlow is a popular branching model that separates the development process into distinct branches. It involves using feature branches for new functionality, a
develop
branch for integrating features, and amaster
branch for production-ready code. Additionally, release and hotfix branches are created for managing software releases and critical bug fixes. - Feature Branching: This strategy involves creating a new branch for each feature or bug fix. It isolates work until it’s ready to be merged into the main branch. Feature branching promotes parallel development and helps avoid conflicts by keeping features separate until they are fully developed.
Both GitFlow and feature branching allow teams to manage code changes systematically, ensuring that features or bug fixes are tested before being integrated into the main codebase.
5. Regular Code Reviews and Merging Caution
Code reviews are a critical part of any development process, as they ensure that only high-quality, bug-free code makes it into the main project. Conducting regular code reviews promotes collaboration, improves code quality, and ensures adherence to coding standards. Additionally, merging branches should always be done with caution.
Best Practices for Code Reviews:
- Be Constructive: When reviewing code, focus on providing constructive feedback rather than criticism. Offer suggestions for improvement and clarify why certain changes are necessary.
- Use Pull Requests: Pull requests (PRs) facilitate code reviews by enabling team members to review changes before they are merged into the main branch. This process ensures that all code changes are evaluated for quality, functionality, and adherence to standards.
- Merge Carefully: Avoid merging branches too hastily. Ensure that the branch is thoroughly tested and that no conflicts exist. If conflicts do arise, resolve them carefully and involve the relevant team members to avoid introducing bugs.
Merging Considerations:
- Merge vs. Rebase: Decide whether to merge or rebase branches based on the team’s workflow. Merging preserves the branch history, while rebasing provides a cleaner, linear history.
- Test Before Merging: Always run tests to ensure the code works as expected before merging. This minimizes the risk of introducing bugs into the main project.
6. Using Tags and Releases for Version Management
Version management is essential for tracking releases and ensuring that the right version of the software is deployed. Git tags allow developers to mark specific points in the commit history, typically used to denote release versions.
Tagging Best Practices:
- Semantic Versioning: Use semantic versioning (e.g., v1.2.3) for your tags. This versioning system provides a clear and structured way of managing releases. It includes three numbers: major, minor, and patch. Major versions introduce breaking changes, minor versions add functionality in a backward-compatible manner, and patch versions contain bug fixes.
- Tagging on Stable Commits: Tag releases only on stable commits that have passed testing and are ready for deployment. Avoid tagging in-progress work or unstable versions.
Tags allow teams to track specific versions of the software, making it easier to revert to a previous version if needed and to deploy the correct version to production.
7. CI/CD Pipelines for Automated Testing and Deployment
Continuous Integration (CI) and Continuous Deployment (CD) pipelines are integral to modern software development. These automated workflows ensure that code is tested and deployed efficiently, reducing the chances of human error.
Benefits of CI/CD Pipelines:
- Automated Testing: CI pipelines run automated tests every time code is committed or merged. This ensures that bugs are caught early and that new changes don’t break existing functionality.
- Automated Deployment: CD pipelines streamline the deployment process by automatically deploying code to staging or production environments after successful testing. This reduces manual intervention and accelerates release cycles.
By integrating CI/CD into the version control process, development teams can enhance code quality, reduce deployment errors, and deliver software faster.
8. Maintaining Documentation for Version Control Processes
Documentation is essential for ensuring that version control best practices are followed consistently across the team. Clear documentation provides guidelines on branch naming conventions, commit message formats, and the overall version control strategy. This helps onboard new developers and maintain consistency in the development process.
Key Areas to Document:
- Branching Strategy: Outline the team’s preferred branching model (e.g., GitFlow) and when to create feature branches, release branches, and hotfixes.
- Commit Message Guidelines: Specify the format for commit messages, including whether to use a ticket number, the imperative mood, and character limits.
- Versioning Conventions: Define how versions are tagged and how releases are managed using semantic versioning.
Having detailed version control documentation ensures that everyone on the team follows the same procedures, reducing confusion and preventing mistakes.
Follow Best Practices
Effective version control is the backbone of successful custom software development. By following best practices such as using Git for code management, crafting clear commit messages, maintaining a clean commit history, and leveraging branching strategies, teams can collaborate more efficiently and manage code changes seamlessly. Regular code reviews, careful merging, and using CI/CD pipelines ensure that high-quality code is integrated into the project and deployed efficiently. Finally, maintaining documentation for version control processes fosters consistency and streamlines onboarding for new team members. By adhering to these version control best practices, development teams can stay organized, reduce errors, and deliver robust custom software on time and with high quality.
You may also be interested in: ALM vs DevOps: Differences in Software Delivery – StudioLabs
Ready to elevate your brand and transform your vision to digital solutions? Since 2003, StudioLabs has been trusted to help conceive, create, and produce digital products for the world’s most well-known brands to cutting-edge startups. Partner with StudioLabs and create something phenomenal. Let’s chat and experience StudioLabs in action with a complimentary session tailored to your business needs!